WordPress is a great platform for building websites. You can use it to create anything from a basic blog or simple business website, to a complex e-commerce store with a shopping cart and online payment options. An important feature of WordPress is that you can extend its functionality through plugins.
There are thousands of plugins available from third-party developers, but there are reasons to write your own. Maybe an existing plugin doesn’t quite do what you need, or hasn’t been updated in a long time. In this article we’ll cover the very basics on how to create a custom plugin for your WordPress site.
Basic Concepts
Step 1: Create the WordPress file
Creating a WordPress plugin requires a folder and a single file. Create a new folder for your plugin called “myplugin” and put it inside the WordPress “wp-content/plugins” folder. Next, create a new file using your favorite plain text editor and add the following code to the top of it:
<?php
/**
* Plugin Name: Name of your plugin
* Plugin URI: URL to the plugin
* Description: A brief summary of what the plugin does
* Version: Version number for this release
* Author: Your name or company name
* Author URI: URL to your personal or corporate website
*/
?>
Replace the text after the colon on each line with information specific to your plugin. For example:
* Plugin Name: My Awesome Plugin
The header fields in this file give WordPress information about the custom plugin. Plugin Name is the only required header field, but typically you’ll include additional fields so users can know more about the plugin, who wrote it, and what it does.
Save the file as “myplugin.php” and make sure it’s inside the “myplugin” folder. Since WordPress is written in PHP, remember to include a “php” file extension.
You now have a valid WordPress plugin that can be installed and activated on a WordPress website. Let’s make it do something.
Step 2: Add functionality through code
The amount of customization available through WordPress plugins is nearly endless. Plugins can add features to the authoring interface, modify the content on posts and pages, create completely new types of posts, and more!
This new functionality is added through hooks. Hooks allow your custom code to interact with WordPress core. There are hundreds of built-in hooks available and you can even create your own. Hooks come in 2 varieties: actions and filters.
Actions add data or change the operation of the WordPress site.
Filters modify data on the WordPress site.
To use action and filter hooks, you’ll need to create a callback function in your custom plugin and then attach (or hook) it to an action or filter. This will run your function when the hook is run.
For example, you can add an action to the “init” hook to set up or configure your plugin. You might want to get user information, load a taxonomy, intercept GET or POST data, or do any other tasks needed for the plugin to work. Let’s add an action to your custom plugin.
function myplugin_config() {
// do stuff to initialize the plugin
}
add_action('init', 'myplugin_config');
Adding a filter is similar. You’ll create a callback function and then attach (or hook) it to a filter.
function myplugin_filter_title($title) {
// do stuff to change the title and return the result
return $title;
}
add_filter('the_title', 'myplugin_filter_title');
Notice the difference? Actions use the “add_action” function to attach your callback function and filters use the “add_filter” function to attach your callback filter. Also, filter callback functions return a result, but action callback functions do not return anything. You’ll typically use a combination of action and filter hooks in your custom plugin.
Step 3: Test and Deploy
As you add more and more features to your custom plugin, it’s important to frequently test the features and check for bugs, performance problems, and security issues.
Developing and testing a custom plugin is much easier if you have multiple environments, say a development, test, and production version of the site. That way, changes can be made in the development or test environments without risking the production environment.
When you’re satisfied that the custom plugin is ready to deploy, you can move it to the production environment with minimal downtime. It’s also a good idea to have a backup of the production site before installing the custom plugin in case you need to revert back to the previous version of the site.
A Custom Plugin
We created a custom WordPress plugin to display a timeline of events. Sure, there are other timeline plugins out there, but none had the right combination of functionality and ease-of-use that our clients wanted.
Our timeline plugin creates a custom post type, aggregates those posts, and displays them in chronological order using a WordPress shortcode. The plugin has configurable settings for colors and sort order, and the timeline display is responsive.
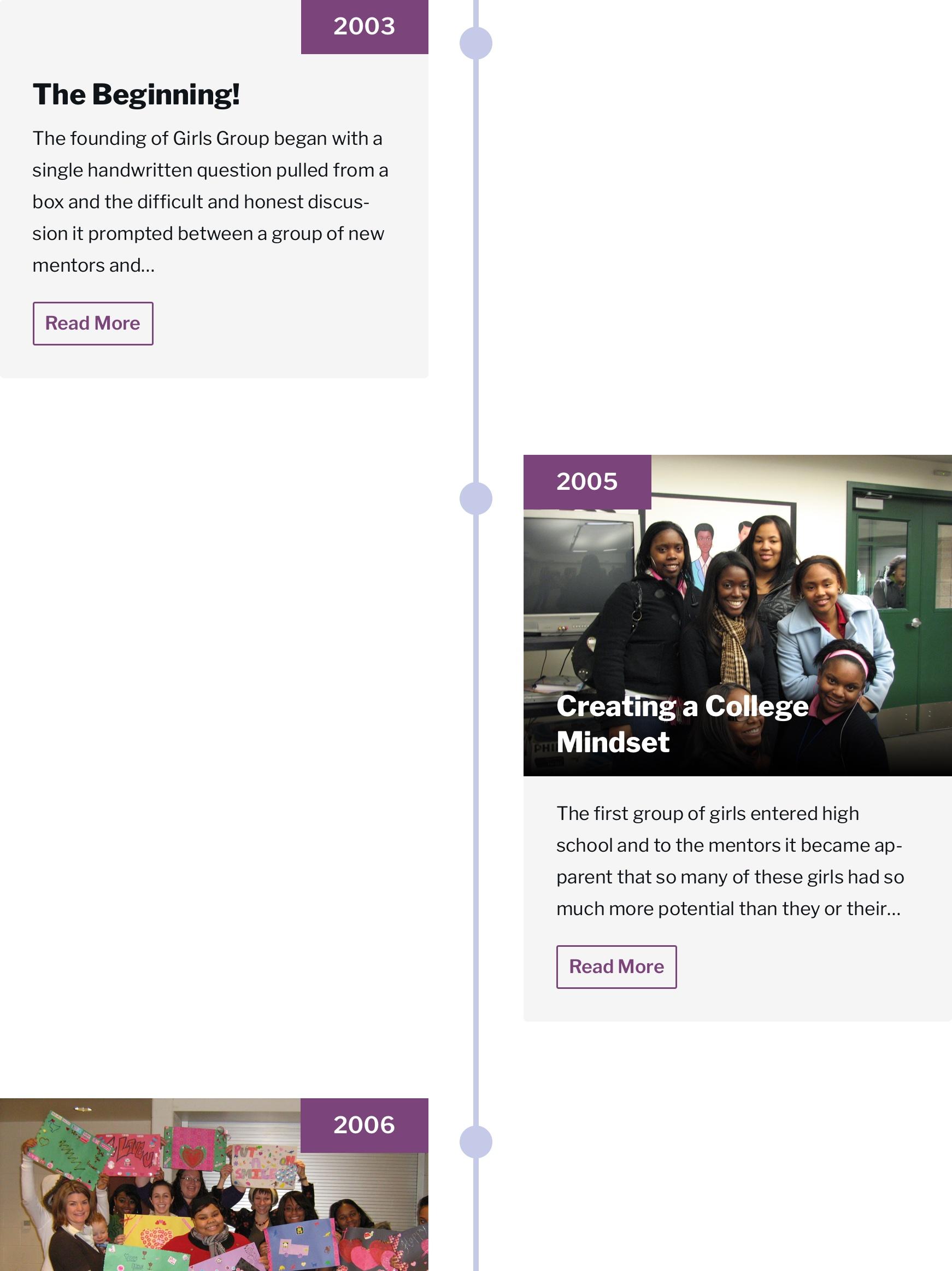
We’ll use features of the Mouko timeline plugin as examples in upcoming articles covering topics such as creating and listing custom post types, using WordPress shortcodes, the administrative user interface, naming conventions, security, coding standards, and more.
Conclusion
This article is an introduction to the basic concepts involved in creating a custom WordPress plugin, but it’s just the tip of the iceberg. We’ll explore many more topics in a series of articles that show the development process from start to finish.
- WordPress
- PHP
- CSS